import java.io.File;
import java.io.IOException;
/*
* 文件操作:创建、查看文件相关信息、删除
*/
public class FileMethods {
public void createFile(File file) {
if (!file.exists()) {
try {
file.createNewFile();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
System.out.println("文件创建成功");
}
}
// 查看文件相关信息
public void showFileInfo(File file) {
if (file.exists()) {
if (file.isFile()) {
// 是文件
System.out.println("文件名" + file.getName());
System.out.println("文件绝对路径" + file.getAbsolutePath());
System.out.println("文件相对路径" + file.getPath());
System.out.println("文件大小" + file.length());
}
if (file.isDirectory()) {
// 是目录
System.out.println("这是一个目录");
}
}
}
//删除文件
public void deleteFile(File file ){
if (file.exists()) {
System.out.println("文件删除成功");
}
}
public static void main(String[] args) {
FileMethods fm=new FileMethods();
File file=new File("D:\\701\\hello.txt");
fm.createFile(file);
//查看文件信息
fm.showFileInfo(file);
}
}
java文件操作
原创
©著作权归作者所有:来自51CTO博客作者晴天MZ的原创作品,请联系作者获取转载授权,否则将追究法律责任
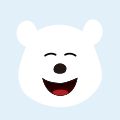
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
.java文件 java文件操作
Java 操作文件包括了两个层面:• 在文件系统的层面上来操作文件,包括创建文件、删除文件、创建目录、拷贝文件等等。• 操作文件里保存的数据,包括读文件、写文件。
java jvm servlet 数据 构造方法 -
java 文件操作 java文件操作实验报告
北京电子科技学院(BESTI) 实 验 报 告 课程:Java 班级: 1352 姓名:黄晓妍 学号:2013
java 文件操作 Java java System -
centos引导报错
一、安装win7和centos7双系统1、 从win7的硬盘中分出20G,用于安装centos7;分出的20G硬盘一定要在windows7的磁盘管理中对其“删除卷”,这样在安装centos7时才可识别出这20G的硬盘,并将centos安装到这20G中,否则无法找到含有NTFS格式的20G硬盘。2、 从centos官网下载CentOS-7-x86_64-DVD-1503-01
centos引导报错 centos7 win7 32位双系统 grub2引导win7 centos 启动项