import java.text.DateFormat; import java.text.ParseException; import java.text.ParsePosition; import java.text.SimpleDateFormat; import java.util.ArrayList; import java.util.Calendar; import java.util.Date; import java.util.GregorianCalendar; import java.util.HashMap; import java.util.LinkedHashMap; import java.util.List; import java.util.Locale; import java.util.Map; import java.util.Random; import java.security.MessageDigest; import java.security.NoSuchAlgorithmException; import org.apache.commons.collections.map.LinkedMap; public final class DateUtil{ public static String getCurrentmin() { SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMddHHmmss"); return sdf.format(new Date()); } /** * 产生随机的2位数 * * @return */ public static String getTwo() { Random rad = new Random(); String result = rad.nextInt(100) + ""; if (result.length() == 1) { result = "0" + result; } return result; } public static String getCurrentH() { SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMddHH"); return sdf.format(new Date()); } public static String getCurrentYMD() { SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd"); return sdf.format(new Date()); } public static String getCurrentDateTime() { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); return sdf.format(new Date()); } public static String getCurrentDateTime2() { return String.valueOf(new Date().getTime()); } public static String getCurrentDate() { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); return sdf.format(new Date()); } public static String getStringDate(Date date) { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); return sdf.format(date); } public static String getCurrentStringDatePremonth() { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); Calendar calendar = Calendar.getInstance(); calendar.add(Calendar.MONTH, -1); return sdf.format(calendar.getTime()); } public static Date getCurrentDatePremonth() { Calendar calendar = Calendar.getInstance(); calendar.add(Calendar.MONTH, -1); return calendar.getTime(); } public static String getCurrentStringDatePreyear() { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); Calendar calendar = Calendar.getInstance(); calendar.add(Calendar.YEAR, -1); return sdf.format(calendar.getTime()); } public static Date getCurrentDatePreyear() { Calendar calendar = Calendar.getInstance(); calendar.add(Calendar.YEAR, -1); return calendar.getTime(); } public static String getCurrentStringDatePreweek() { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); Calendar calendar = Calendar.getInstance(); calendar.add(Calendar.WEEK_OF_YEAR, -1); return sdf.format(calendar.getTime()); } public static Date getCurrentDatePreweek() { Calendar calendar = Calendar.getInstance(); calendar.add(Calendar.WEEK_OF_YEAR, -1); return calendar.getTime(); } public static String getAfterTime(int min) { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); Calendar nowTime = Calendar.getInstance(); nowTime.add(Calendar.MINUTE, min); return sdf.format(nowTime.getTime()); } public static String parseDate(String date) { SimpleDateFormat sdf = new SimpleDateFormat("yyyy年MM月dd日"); Date date2 = null; try { date2 = sdf.parse(date); } catch (ParseException e) { return ""; } return sdf.format(date2); } public static Date parseDate(String source, String pattern) { SimpleDateFormat format = new SimpleDateFormat(pattern); try { return format.parse(source); } catch (ParseException e) { } return null; } public static String parseDate(Date date, String pattern) { SimpleDateFormat format = new SimpleDateFormat(pattern); try { return format.format(date); } catch (Exception e) { } return null; } public static String formatTime(String time) { try { Date date = new Date(Long.parseLong(time)); Date currentTime = new Date(); SimpleDateFormat sdf = null; if (getYear(date) == getYear(currentTime)) { if ((getMonth(date) == getMonth(currentTime)) && (getDay(date) == getDay(currentTime))) { sdf = new SimpleDateFormat("HH:mm"); return sdf.format(date); } sdf = new SimpleDateFormat("M月d日"); return sdf.format(date); } sdf = new SimpleDateFormat("yyyy年M月d日"); return sdf.format(date); } catch (Exception e) { } return ""; } public static String formatTime2(String time) { try { Date date = new Date(Long.parseLong(time)); Date currentTime = new Date(); SimpleDateFormat sdf = null; if (getYear(date) == getYear(currentTime)) { sdf = new SimpleDateFormat("M月d日 HH:mm"); return sdf.format(date); } sdf = new SimpleDateFormat("yyyy年M月d日 HH:mm"); return sdf.format(date); } catch (Exception e) { } return ""; } public static int getMonthMaxDay(String date) throws ParseException { return getMonthMaxDay(formatDate(date)); } public static int getMonthMaxDay(Date date) { Calendar c = setCalendar(date); return c.getActualMaximum(5); } public static int getYear(Date date) { return setCalendar(date).get(1); } public static int getMonth(Date date) { return setCalendar(date).get(2) + 1; } public static int getDay(Date date) { return setCalendar(date).get(5); } public static int getWeek(Date date) { return setCalendar(date).get(7) - 1; } public static int getHours(Date date) { return setCalendar(date).get(11); } public static int getMinutes(Date date) { return setCalendar(date).get(12); } public static int getSeconds(Date date) { return setCalendar(date).get(13); } public static Date getWeekByNum(Date date, int num) { Calendar c = setCalendar(date); c.set(7, num); return c.getTime(); } public static Calendar setCalendar(Date date) { Calendar c = Calendar.getInstance(Locale.CHINA); c.setTime(date); return c; } public static Date setCalendar(Date date, int day) { Calendar c = setCalendar(date); c.set(5, day); return c.getTime(); } public static Date addDay(Date date, int num) { Calendar c = setCalendar(date); c.add(5, num); return c.getTime(); } public static Date addMonth(Date date, int num) { Calendar c = setCalendar(date); c.add(2, num); return c.getTime(); } public static Date setTime(Date date, int hour, int minute, int second) { Calendar c = setCalendar(date); c.set(11, hour); c.set(12, minute); c.set(13, second); return c.getTime(); } public static Date set(Date date, int pattern, int param) { Calendar c = setCalendar(date); c.set(pattern, param); return c.getTime(); } public static Date formatDate(String date, String pattern) throws ParseException { if ((pattern == null) || ("".equals(pattern))) { return formatDate(date); } SimpleDateFormat sdf = new SimpleDateFormat(pattern); return sdf.parse(date); } public static Date formatDate(String date) throws ParseException { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); return sdf.parse(date); } public static Date formatDate(Object obj) throws ParseException { if ((obj instanceof Date)) { return (Date) obj; } throw new ParseException("convert is error", 1); } public static String toString(Date date, String pattern) { SimpleDateFormat sdf = new SimpleDateFormat(pattern); return sdf.format(date); } public static String toString(Date date) { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); return sdf.format(date); } public static String toYYYYMMDDStr(Date date) { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); return sdf.format(date); } public static Date getTodayDate() { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); try { return sdf.parse(getCurrentDateTime()); } catch (ParseException e) { e.printStackTrace(); } return null; } public static String getYseterday() { Calendar cal = Calendar.getInstance(); cal.add(Calendar.DATE, -1); return new SimpleDateFormat("yyyy-MM-dd ").format(cal.getTime()); } public static Date getCurrentTime() { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); try { return sdf.parse(getCurrentDateTime()); } catch (ParseException e) { e.printStackTrace(); } return null; } public static Date add(Date date, int field, int num) { Calendar c = setCalendar(date); c.add(field, num); return c.getTime(); } public static int getWeekOfYear(Date date) { Calendar c = new GregorianCalendar(); c.setFirstDayOfWeek(2); c.setMinimalDaysInFirstWeek(7); c.setTime(date); return c.get(3); } public static int getMaxWeekNumOfYear(int year) { Calendar c = new GregorianCalendar(); c.set(year, 11, 31, 23, 59, 59); return getWeekOfYear(c.getTime()); } public static Date getFirstDayOfWeek(int year, int week) { Calendar c = new GregorianCalendar(); c.set(1, year); c.set(2, 0); c.set(5, 1); Calendar cal = (GregorianCalendar) c.clone(); cal.add(5, week * 7); return getFirstDayOfWeek(cal.getTime()); } public static Date getLastDayOfWeek(int year, int week) { Calendar c = new GregorianCalendar(); c.set(1, year); c.set(2, 0); c.set(5, 1); Calendar cal = (GregorianCalendar) c.clone(); cal.add(5, week * 7); return getLastDayOfWeek(cal.getTime()); } public static Date getFirstDayOfWeek(Date date) { Calendar c = new GregorianCalendar(); c.setFirstDayOfWeek(2); c.setTime(date); c.set(7, c.getFirstDayOfWeek()); return c.getTime(); } public static Date getLastDayOfWeek(Date date) { Calendar c = new GregorianCalendar(); c.setFirstDayOfWeek(2); c.setTime(date); c.set(7, c.getFirstDayOfWeek() + 6); return c.getTime(); } public static Date getFirstDayOfWeek() { Calendar c = new GregorianCalendar(); c.setFirstDayOfWeek(2); c.setTime(new Date()); c.set(7, c.getFirstDayOfWeek()); return c.getTime(); } public static Date getLastDayOfWeek() { Calendar c = new GregorianCalendar(); c.setFirstDayOfWeek(2); c.setTime(new Date()); c.set(7, c.getFirstDayOfWeek() + 6); return c.getTime(); } public static Date getFirstDayOfMonth() { Calendar c = new GregorianCalendar(); c.setFirstDayOfWeek(2); c.setTime(new Date()); c.set(5, 1); return c.getTime(); } public static Date getLastDayOfMonth() { Calendar c = new GregorianCalendar(); c.setFirstDayOfWeek(2); c.setTime(new Date()); c.set(5, getMonthMaxDay(new Date())); return c.getTime(); } public static Date getFirstDateOfCurrentMonth() { Date lastMonth = addMonth(new Date(), 0); Date lastDate = setCalendar(lastMonth, 1); return setTime(lastDate, 0, 0, 0); } public static Date getLastDateOfCurrentMonth() { Date lastMonth = addMonth(new Date(), 0); int max = getMonthMaxDay(lastMonth); Date lastDate = setCalendar(lastMonth, max); return setTime(lastDate, 23, 59, 59); } public static Date getFirstDateOfLastMonth() { Date lastMonth = addMonth(new Date(), -1); Date lastDate = setCalendar(lastMonth, 1); return setTime(lastDate, 0, 0, 0); } public static Date getLastDateOfLastMonth() { Date lastMonth = addMonth(new Date(), -1); int max = getMonthMaxDay(lastMonth); Date lastDate = setCalendar(lastMonth, max); return setTime(lastDate, 23, 59, 59); } public static Date getFirstDateOfBeforeThreeMonth() { Date lastMonth = addMonth(new Date(), -3); Date lastDate = setCalendar(lastMonth, 1); return setTime(lastDate, 0, 0, 0); } public static Date getFirstDateOfLastDate() { Date date = addDay(new Date(), -1); return setTime(date, 0, 0, 0); } public static Date getLastDateOfLastDate() { Date date = addDay(new Date(), -1); return setTime(date, 23, 59, 59); } public static Date getFirstDateOfLastHour() { Date now = new Date(); int hour = getHours(now) - 1; Date date = null; if (hour == -1) { date = addDay(now, -1); hour = 23; } else { date = now; } return setTime(date, hour, 0, 0); } public static Date getLastDateOfLastHour() { Date now = new Date(); int hour = getHours(now) - 1; Date date = null; if (hour == -1) { date = addDay(now, -1); hour = 23; } else { date = now; } return setTime(date, hour, 59, 59); } public static long dateDiff(Date dateStart, Date dateEnd) { return dateEnd.getTime() - dateStart.getTime(); } public static int getDiffDay(Date begin_date, Date end_date) { int day = 0; try { SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd"); String sdate = format.format(Calendar.getInstance().getTime()); if (begin_date == null) { begin_date = format.parse(sdate); } if (end_date == null) { end_date = format.parse(sdate); } day = (int) ((end_date.getTime() - begin_date.getTime()) / (24 * 60 * 60 * 1000)); } catch (Exception e) { return -1; } return day; } public static boolean isDate(String date) { try { if ((date == null) || ("".equals(date)) || (!date.matches("[0-9]{8}"))) { return false; } int year = Integer.parseInt(date.substring(0, 4)); int month = Integer.parseInt(date.substring(4, 6)) - 1; int day = Integer.parseInt(date.substring(6)); Calendar calendar = GregorianCalendar.getInstance(); calendar.setLenient(false); calendar.set(1, year); calendar.set(2, month); calendar.set(5, day); calendar.get(1); } catch (IllegalArgumentException e) { return false; } return true; } public static List<Date> getDates(Calendar p_start, Calendar p_end) { List<Date> result = new ArrayList<Date>(); while (!p_start.after(p_end)) { result.add(p_start.getTime()); p_start.add(Calendar.DAY_OF_YEAR, 1); } return result; } /** * 将时间转成这种格式 * * @param date * - string类型的格式化时间 * @param format1 * - 原本的时间格式 * @param format2 * - 装换后的时间格式 * @return */ public static String strToFormat(String date, String format1, String format2) { SimpleDateFormat formatter1 = new SimpleDateFormat(format1); SimpleDateFormat formatter2 = new SimpleDateFormat(format2); String dateString = null; try { dateString = formatter2.format(formatter1.parse(date)); } catch (ParseException e) { // TODO Auto-generated catch block e.printStackTrace(); } return dateString; } /** * 将短时间格式字符串转换为时间 yyyy-MM-dd HH:mm:ss * * @param strDate * @return */ public static Date strToDate(String strDate) { SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); ParsePosition pos = new ParsePosition(0); Date strtodate = formatter.parse(strDate, pos); return strtodate; } /** * 比较两个字符串类型的日期相差多少天 * * @param date1 * @param date2 * @param patten * @return */ public static int compareSDateNum(String date1, String date2, String patten) { try { SimpleDateFormat sdf = new SimpleDateFormat(patten); Calendar cal = Calendar.getInstance(); cal.setTime(sdf.parse(date1)); long time1 = cal.getTimeInMillis(); cal.setTime(sdf.parse(date2)); long time2 = cal.getTimeInMillis(); long between_days = (time2 - time1) / (1000 * 3600 * 24); return Integer.parseInt(String.valueOf(between_days)); } catch (ParseException e) { e.printStackTrace(); return 0; } } /** * 获取24小时前 */ public static String getBefore24Hour() { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); Calendar calendar = Calendar.getInstance(); calendar.add(Calendar.HOUR_OF_DAY, -24); return sdf.format(calendar.getTime()); } /** * 获取24小时后 */ public static String getAfter24Hour() { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); Calendar calendar = Calendar.getInstance(); calendar.add(Calendar.HOUR_OF_DAY, 24); return sdf.format(calendar.getTime()); } /** * 比较两个字符串类型的日期 * * @param date1 * @param date2 * @param patten * @return */ public static long compareSDate(String date1, String date2, String patten) { try { SimpleDateFormat sdf = new SimpleDateFormat(patten); Calendar cal = Calendar.getInstance(); cal.setTime(sdf.parse(date1)); long time1 = cal.getTimeInMillis(); cal.setTime(sdf.parse(date2)); long time2 = cal.getTimeInMillis(); return time1 - time2; } catch (ParseException e) { e.printStackTrace(); return 0; } } /** * 比较两个字符串类型的日期 * * @param date1 * @param date2 * @param patten * @return */ public static long compareSDate(String date1, String date2) { try { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); Calendar cal = Calendar.getInstance(); cal.setTime(sdf.parse(date1)); long time1 = cal.getTimeInMillis(); cal.setTime(sdf.parse(date2)); long time2 = cal.getTimeInMillis(); return time1 - time2; } catch (ParseException e) { e.printStackTrace(); return 0; } } /** * 获取当天是星期几 */ public static int getCurrentDayToWeek() { Calendar cal = Calendar.getInstance(); cal.setTime(new Date()); return cal.get(Calendar.DAY_OF_WEEK); } /** * 判断当前时间是上午还是下午 */ public static boolean getAmOrPm() { Calendar cal = Calendar.getInstance(); cal.setTime(new Date()); return cal.get(Calendar.AM_PM) == 0; } /** * 星期转为日期 */ public static List<LinkedHashMap<String, Object>> weekToDateString( String startDate, String endDate) { int start = Integer.parseInt(startDate); int end = Integer.parseInt(endDate); List<LinkedHashMap<String, Object>> dateStr = new ArrayList<LinkedHashMap<String, Object>>(); for (int i = 1; i <= 7; i++) { Calendar c = Calendar.getInstance(); c.add(Calendar.DATE, i); if (start <= c.get(Calendar.DAY_OF_WEEK) && end >= c.get(Calendar.DAY_OF_WEEK)) { LinkedHashMap<String, Object> map = new LinkedHashMap<String, Object>(); map.put("date", toYYYYMMDDStr(c.getTime())); map.put("week", c.get(Calendar.DAY_OF_WEEK)); dateStr.add(map); } } return dateStr; } // 去掉小数点后面的 public static String getNumber(String str) { if (str.indexOf(".") < 0) { return str; } return str.substring(0, str.indexOf(".")); } // 日期比较 public static int compare_date(String DATE1, String DATE2) { DateFormat df = new SimpleDateFormat("yyyy-MM-dd"); try { Date dt1 = df.parse(DATE1); Date dt2 = df.parse(DATE2); if (dt1.getTime() > dt2.getTime()) { System.out.println("dt1 在dt2前"); return 1; } else if (dt1.getTime() < dt2.getTime()) { System.out.println("dt1 在dt2后"); return -1; } else { return 0; } } catch (Exception exception) { exception.printStackTrace(); } return 0; } // 当前时间 public static String gettime() { Date dt = new Date(); SimpleDateFormat matter1 = new SimpleDateFormat("yyyy-MM-dd"); return matter1.format(dt); } // 明天的时间 public static String gettime1() { Date date = new Date(); Calendar calendar = new GregorianCalendar(); calendar.setTime(date); calendar.add(calendar.DATE, 1);// 把日期往后增加一天.整数往后推,负数往前移动 date = calendar.getTime(); // 这个时间就是日期往后推一天的结果 SimpleDateFormat matter1 = new SimpleDateFormat("yyyy-MM-dd"); return matter1.format(date); } }
时间工具类DateUtil
精选 转载上一篇:文件缓存FileCache
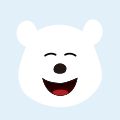
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章