Spring学习笔记(二)
原创
©著作权归作者所有:来自51CTO博客作者shirly51211的原创作品,谢绝转载,否则将追究法律责任
一、基本装配(IOC的基本使用)
(1)、setter方式(必须依靠无参构造器)
A、基本类型(8中基本类型+字符串)的装配
B、对象类型的装配
C、集合的装配
A、基本类型的装配
注意: 用setter方式注入的话,必须要用set、get方法
例子:
public class HelloBean {
private String name;
private int age;
public String sayHello(){
return \"hello \"+name +\",your age is\" + age;
}
.............
}
配置文件applicationContext.xml
<bean id=\"helloBean\" class=\"ioc1.HelloBean\">
<property name=\"name\">
<value>lishi</value>
</property>
<property name=\"age\" value=\"20\">
</property>
</bean>
<!--id是Bean的唯一标识,要求在整个配置文件中要唯一-->
<!--property 对于所有用setter方式来注入的必须用Property来指定-->
<!--value 是对以基本类型,都用value来注入,可以实现自动的数据类型转换-->
测试类:
public class Test {
public static void main(String[] args) {
ApplicationContext ac =
new ClassPathXmlApplicationContext(\"ioc1\\\\applicationContext.xml\");
//获取容器的一个实例
HelloBean hb = (HelloBean) ac.getBean(\"helloBean\");
System.out.println(hb.sayHello());
}
}
B、对象类型的装配
(1)、<ref local=\" \"/> 用于涉及的对象的id在本配置文件中
(2)、<ref bean=\" \"/> 用于涉及的对象的id不再本配置文件中
(3)、嵌套
public class OtherBean {
private String str1;
public String getStr1() {
return str1;
}
public void setStr1(String str1) {
this.str1 = str1;
}
public String toString(){
return \"OtherBean \"+str1;
}
}
public class SomeBean {
private OtherBean ob;
public void printInfo(){
System.out.println(\"someBean \"+ob);
}
public OtherBean getOb() {
return ob;
}
public void setOb(OtherBean ob) {
this.ob = ob;
}
}
配置applicationContext.xml
<bean id=\"someBean\" class=\"ioc2.SomeBean\">
<property name=\"ob\">
<ref bean=\"otherBean\" />
</property>
</bean>
配置other.xml文件
<bean id=\"otherBean\" class=\"ioc2.OtherBean\">
<property name=\"str1\">
<value>string1</value>
</property>
</bean>
测试类:
public static void main(String[] args) {
ApplicationContext ac = new ClassPathXmlApplicationContext(new String[]{\"ioc2//applicationContext.xml\",\"ioc2//other.xml\"});
SomeBean sb = (SomeBean) ac.getBean(\"someBean\");
sb.printInfo();
}
上一篇:Spring学习笔记(一)
下一篇:Spring学习笔记(三)
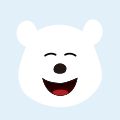
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【CAD建模号】学习笔记(二):工作区
工作区由[工具提示],[自适应网格],[自适应坐标轴],[参考坐标轴],[绘制的图形]组成。
自适应 坐标轴 工具提示 工作区 CAD建模号 -
Spring学习笔记AOP(二)
Spring学习笔记AOP(二)基于注解的AOP
Java AspectJ -
Spring Boot学习笔记(二)
1.Spring Boot配置文件
spring boot spring html 配置文件 -
Spring学习笔记(二)Servlet基础
Spring学习笔记(二)Servlet基础
httpservlet