#include<stdio.h> typedef enum Tag { C_ANNOTAION_START = 0, // C注释的开始 C_ANNOTAION_END = 1, // C注释的结束 }Tag; typedef enum State { SUCCESS = 1,//成功 OPEN_FILE_ERROR,//打开文件失败 NO_MATCH,//文档不匹配 OTHER,//其他 }State; State AnnotationConvert(FILE* cIn,FILE* cOut ) { char FirstCh, SecondCh; Tag Is_Start = C_ANNOTAION_END;//是否开始转换 do { FirstCh = fgetc(cIn); switch (FirstCh) { // 1.一般情况 ///* int i = 0; */ case '/': SecondCh = fgetc(cIn); // 3.匹配问题 ///*int i = 0;/*xxxxx*/ if (SecondCh == '*'&&Is_Start == C_ANNOTAION_END) { fputc('/', cOut); fputc('/', cOut); Is_Start = C_ANNOTAION_START; } else if (SecondCh == '/')//c++注释问题 { char Next = fgetc(cIn); fputc('/', cOut); fputc('/', cOut); while (Next != '\n'&&Next!=EOF) { fputc(Next, cOut); Next = fgetc(cIn); } //FirstCh = Next; fseek(cIn, -1, SEEK_CUR); } else { fputc(FirstCh, cOut); fputc(SecondCh, cOut); } break; case '*': SecondCh = fgetc(cIn); // 2.换行问题/5.连续注释问题 ///* int i = 0; */int j = 0; ///* int i = 0; */ if (SecondCh == '/') { char Next = 0; Next = fgetc(cIn); if (Next != '\n'&&Next != EOF) { fseek(cIn, -1, SEEK_CUR); fputc('\n', cOut); } else { fputc('\n', cOut); } Is_Start = C_ANNOTAION_END; } else if (SecondCh == '*') { fputc(FirstCh,cOut); fseek(cIn, -1, SEEK_CUR); break; } else { fputc(FirstCh, cOut); fputc(SecondCh, cOut); } break; // 4.多行注释问题 case '\n': fputc('\n', cOut); if (Is_Start == C_ANNOTAION_START) { fputc('/', cOut); fputc('/', cOut); } break; default: fputc(FirstCh, cOut); break; } } while (FirstCh != EOF); if (Is_Start == C_ANNOTAION_START) { return NO_MATCH; } return SUCCESS; } State StartAnnotationConvert(const char * input, const char * output) { FILE* cIn = fopen(input, "r"); FILE* cOut = fopen(output, "w"); State ret = 0; if (cIn == NULL) { fclose(cOut); return OPEN_FILE_ERROR; } if (cOut == NULL) { fclose(cIn); return OPEN_FILE_ERROR; } ret=AnnotationConvert(cIn, cOut); fclose(cIn); fclose(cOut); return ret; } int main() { State ret = StartAnnotationConvert("input.c", "output.c"); if (ret == SUCCESS) { printf("转换成功!\n"); } else if (ret ==NO_MATCH) { printf("文档未找到匹配结尾\n"); } else if (ret == OPEN_FILE_ERROR) { printf("打开文件失败\n"); } return 0; }
c语言 c到c++的注释转换
原创文章标签 c;文件操作;注释转换 文章分类 C/C++ 后端开发
上一篇:c语言 将字符串转化为整数
下一篇:c 语言 静态顺序表 基本操作
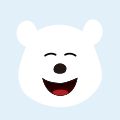
-
C语言实现C到C++的注释转换
C语言实现C到C++的注释转换
C语言 注释转换 -
c语言项目—注释转换(c——c++)
注释转换项目!
c语言 include 测试结果 -
C语言注释转c++注释
将C语言注释转换成c++注释
C/C++ 注释转换 -
C注释 转换为 C++注释
C注释 转换为 C++注释
C注释 转换为   -
C语言简单实现:将C注释转化为C++注释
C语言简单实现:将C注释转化为C++注释
c++ #include #define -
从C语言到C++的进阶之C到C++的转变(篇一)
C++是在C语言的基础上发展出来的,在C语言的基础上又形成了新的对象、类、继承等用法,本文专注
c++ 编程语言 数据结构 经验分享 程序人生