using OpenCvSharp;
using OpenCvSharp.Dnn;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace OPenCVDemo
{
class Program
{
static void Main(string[] args)
{
const string configFile = "deploy.prototxt";
const string faceModel = "res10_300x300_ssd_iter_140000_fp16.caffemodel";
const string image = "3.jpg";
// Read sample image
var frame = Cv2.ImRead(image);
int frameHeight = frame.Rows;
int frameWidth = frame.Cols;
var faceNet = CvDnn.ReadNetFromCaffe(configFile, faceModel);
var blob = CvDnn.BlobFromImage(frame, 1.0, new Size(300, 300), new Scalar(104, 117, 123), false, false);
faceNet.SetInput(blob, "data");
var detection = faceNet.Forward("detection_out");
var detectionMat = new Mat(detection.Size(2), detection.Size(3), MatType.CV_32F,
detection.Ptr(0));
for (int i = 0; i < detectionMat.Rows; i++)
{
float confidence = detectionMat.At<float>(i, 2);
if (confidence > 0.7)
{
int x1 = (int)(detectionMat.At<float>(i, 3) * frameWidth);
int y1 = (int)(detectionMat.At<float>(i, 4) * frameHeight);
int x2 = (int)(detectionMat.At<float>(i, 5) * frameWidth);
int y2 = (int)(detectionMat.At<float>(i, 6) * frameHeight);
Cv2.Rectangle(frame, new Point(x1, y1), new Point(x2, y2), new Scalar(0, 255, 0), 2, LineTypes.Link4);
}
}
Window.ShowImages(frame);
}
}
}
C# OpenCv DNN 人脸检测
原创
©著作权归作者所有:来自51CTO博客作者天天代码码天天的原创作品,请联系作者获取转载授权,否则将追究法律责任
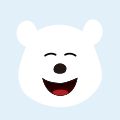
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
OpenCV/DNN 人脸(目标)检测
使用深度学习模块进行公共成员函数#include <opencv2\open
opencv c++ ide #include 人脸检测 -
python opencv DNN 人脸检测
import cv2modelFile = "res10_300x300_ssd_iter_140000_fp16.caffemodel"configFile = "d
opencv python 计算机视觉 ide DNN