package main
import (
"context"
"github.com/go-redis/redis/v8"
"log"
"sync"
)
var ctx = context.Background()
func ProducerMessageList(rdb *redis.Client, queueListKey string) {
for i := 0; i < 100; i++ {
_, err := rdb.Publish(ctx, queueListKey, i).Result()
if err != nil {
log.Println(err)
}
}
}
func ConsumerMessageList(pubSub *redis.PubSub, node int) {
for {
ch := pubSub.Channel()
for msg := range ch {
log.Printf("消费者 %d ------------------ channel:%s;message:%s\n", node, msg.Channel, msg.Payload)
}
}
}
func main() {
rdb := redis.NewClient(&redis.Options{
Addr: "192.168.117.129:6379",
Password: "foobared", // no password set
DB: 0, // use default DB
})
// 测试连接是否成功
_ = rdb.Ping(context.Background()).Err()
go ProducerMessageList(rdb, "group1")
pubSub := rdb.Subscribe(context.Background(), "group1")
_ = pubSub.PSubscribe(context.Background(), "group1:*")
var wg sync.WaitGroup
for i := 0; i < 10; i++ {
wg.Add(1)
go ConsumerMessageList(pubSub, i)
}
wg.Wait()
}
go redis 组订阅 并发 消费
原创
©著作权归作者所有:来自51CTO博客作者go工程师的原创作品,请联系作者获取转载授权,否则将追究法律责任
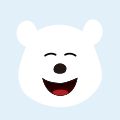
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章