var COREHTML5 = COREHTML5 || {}
// Constructor........................................................
COREHTML5.RoundedRectangle = function(strokeStyle, fillStyle,
horizontalSizePercent,
verticalSizePercent) {
this.strokeStyle = strokeStyle ? strokeStyle : 'gray';
this.fillStyle = fillStyle ? fillStyle : 'skyblue';
horizontalSizePercent = horizontalSizePercent || 100;
verticalSizePercent = verticalSizePercent || 100;
this.SHADOW_COLOR = 'rgba(100, 100, 100, 0.8)';
this.SHADOW_OFFSET_X = 3;
this.SHADOW_OFFSET_Y = 3;
this.SHADOW_BLUR = 3;
this.setSizePercents(horizontalSizePercent,
verticalSizePercent);
this.createCanvas();
this.createDOMElement();
return this;
}
// Prototype..........................................................
COREHTML5.RoundedRectangle.prototype = {
// General functions ..............................................
createCanvas: function () {
var canvas = document.createElement('canvas');
this.context = canvas.getContext('2d');
return canvas;
},
createDOMElement: function () {
this.domElement = document.createElement('div');
this.domElement.appendChild(this.context.canvas);
},
appendTo: function (element) {
element.appendChild(this.domElement);
this.domElement.style.width = element.offsetWidth + 'px';
this.domElement.style.height = element.offsetHeight + 'px';
this.resize(element.offsetWidth, element.offsetHeight);
},
resize: function (width, height) {
this.HORIZONTAL_MARGIN = (width - width *
this.horizontalSizePercent)/2;
this.VERTICAL_MARGIN = (height - height *
this.verticalSizePercent)/2;
this.cornerRadius = (this.context.canvas.height/2 -
2*this.VERTICAL_MARGIN)/2;
this.top = this.VERTICAL_MARGIN;
this.left = this.HORIZONTAL_MARGIN;
this.right = this.left + width - 2*this.HORIZONTAL_MARGIN;
this.bottom = this.top + height - 2*this.VERTICAL_MARGIN;
this.context.canvas.width = width;
this.context.canvas.height = height;
},
setSizePercents: function (h, v) {
// horizontalSizePercent and verticalSizePercent
// represent the size of the rounded rectangle in terms
// of horizontal and vertical percents of the rectangle's
// enclosing DOM element.
this.horizontalSizePercent = h > 1 ? h/100 : h;
this.verticalSizePercent = v > 1 ? v/100 : v;
},
// Drawing Functions...............................................
fill: function () {
var radius = (this.bottom - this.top) / 2;
this.context.save();
this.context.shadowColor = this.SHADOW_COLOR;
this.context.shadowOffsetX = this.SHADOW_OFFSET_X;
this.context.shadowOffsetY = this.SHADOW_OFFSET_Y;
this.context.shadowBlur = 6;
this.context.beginPath();
this.context.moveTo(this.left + radius, this.top);
this.context.arcTo(this.right, this.top,
this.right, this.bottom, radius);
this.context.arcTo(this.right, this.bottom,
this.left, this.bottom, radius);
this.context.arcTo(this.left, this.bottom,
this.left, this.top, radius);
this.context.arcTo(this.left, this.top,
this.right, this.top, radius);
this.context.closePath();
this.context.fillStyle = this.fillStyle;
this.context.fill();
this.context.shadowColor = undefined;
},
overlayGradient: function () {
var gradient =
this.context.createLinearGradient(this.left, this.top,
this.left, this.bottom);
gradient.addColorStop(0, 'rgba(255,255,255,0.4)');
gradient.addColorStop(0.2, 'rgba(255,255,255,0.6)');
gradient.addColorStop(0.25, 'rgba(255,255,255,0.7)');
gradient.addColorStop(0.3, 'rgba(255,255,255,0.9)');
gradient.addColorStop(0.40, 'rgba(255,255,255,0.7)');
gradient.addColorStop(0.45, 'rgba(255,255,255,0.6)');
gradient.addColorStop(0.60, 'rgba(255,255,255,0.4)');
gradient.addColorStop(1, 'rgba(255,255,255,0.1)');
this.context.fillStyle = gradient;
this.context.fill();
this.context.lineWidth = 0.4;
this.context.strokeStyle = this.strokeStyle;
this.context.stroke();
this.context.restore();
},
draw: function (context) {
var originalContext;
if (context) {
originalContext = this.context;
this.context = context;
}
this.fill();
this.overlayGradient();
if (context) {
this.context = originalContext;
}
},
erase: function() {
// Erase the entire canvas
this.context.clearRect(0, 0, this.context.canvas.width,
this.context.canvas.height);
}
};
//......................................................
var widthRange = document.getElementById('widthRange'),
heightRange = document.getElementById('heightRange'),
roundedRectangle = new COREHTML5.RoundedRectangle(
'rgba(0, 0, 0, 0.2)', 'darkgoldenrod',
widthRange.value, heightRange.value);
function resize() {
roundedRectangle.horizontalSizePercent = widthRange.value/100;
roundedRectangle.verticalSizePercent = heightRange.value/100;
roundedRectangle.resize(roundedRectangle.domElement.offsetWidth,
roundedRectangle.domElement.offsetHeight);
roundedRectangle.erase();
roundedRectangle.draw();
}
widthRange.onchange = resize;
heightRange.onchange = resize;
roundedRectangle.appendTo(document.getElementById('roundedRectangleDiv'));
roundedRectangle.draw();
圆角矩形RoundedRectangle46
原创
©著作权归作者所有:来自51CTO博客作者生而为人我很遗憾的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:自定义控件45
下一篇:进度条Progressbar47
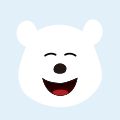
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
51c大模型~合集46
大模型
大模型 -
Android圆角矩形图标 安卓圆角矩形
我们的项目的设计师喜欢用圆角矩形背景作为设计元素,而且颜色、样式各不一样导致项目工程里面定义了大量的xml文件,为了消除这一现象,我想到自定义控件解决这个问题。
Android圆角矩形图标 android 控件 圆角矩形 安卓 -
android arcTo 圆角矩形 android绘制圆角矩形
Android自定义圆角矩形进度条
android arcTo 圆角矩形 android view 自定义 progress -
Flex圆角矩形
Te...
xml 其他 -
android 矩形边框圆角问题 android studio 圆角矩形
用于把普通图片转换为圆角图像的工具类RoundRect类
android 图片圆角 Utils Android android 圆角矩形