var canvas = document.getElementById('canvas'),
context = canvas.getContext('2d'),
eraseAllButton = document.getElementById('eraseAllButton'),
strokeStyleSelect = document.getElementById('strokeStyleSelect'),
fillStyleSelect = document.getElementById('fillStyleSelect'),
fillCheckbox = document.getElementById('fillCheckbox'),
guidewireCheckbox = document.getElementById('guidewireCheckbox'),
drawingSurfaceImageData,
mousedown = {},
rubberbandRect = {},
dragging = false,
guidewires = true;
// General-purpose functions.....................................
function drawGrid(color, stepx, stepy) {
context.save()
context.strokeStyle = color;
context.fillStyle = '#ffffff';
context.lineWidth = 0.5;
context.fillRect(0, 0, context.canvas.width, context.canvas.height);
for (var i = stepx + 0.5; i < context.canvas.width; i += stepx) {
context.beginPath();
context.moveTo(i, 0);
context.lineTo(i, context.canvas.height);
context.stroke();
}
for (var i = stepy + 0.5; i < context.canvas.height; i += stepy) {
context.beginPath();
context.moveTo(0, i);
context.lineTo(context.canvas.width, i);
context.stroke();
}
context.restore();
}
function windowToCanvas(x, y) {
var bbox = canvas.getBoundingClientRect();
return { x: x - bbox.left * (canvas.width / bbox.width),
y: y - bbox.top * (canvas.height / bbox.height)
};
}
// Save and restore drawing surface..............................
function saveDrawingSurface() {
drawingSurfaceImageData = context.getImageData(0, 0,
canvas.width,
canvas.height);
}
function restoreDrawingSurface() {
context.putImageData(drawingSurfaceImageData, 0, 0);
}
// Rubberbands...................................................
function updateRubberbandRectangle(loc) {
rubberbandRect.width = Math.abs(loc.x - mousedown.x);
rubberbandRect.height = Math.abs(loc.y - mousedown.y);
if (loc.x > mousedown.x) rubberbandRect.left = mousedown.x;
else rubberbandRect.left = loc.x;
if (loc.y > mousedown.y) rubberbandRect.top = mousedown.y;
else rubberbandRect.top = loc.y;
}
function drawRubberbandShape(loc) {
var angle,
radius;
if (mousedown.y === loc.y) { // horizontal line
// Horizontal lines are a special case. See the else
// block for an explanation
radius = Math.abs(loc.x - mousedown.x);
}
else {
// For horizontal lines, the angle is 0, and Math.sin(0)
// is 0, which means we would be dividing by 0 here to get NaN
// for radius. The if block above catches horizontal lines.
angle = Math.atan(rubberbandRect.height/rubberbandRect.width),
radius = rubberbandRect.height / Math.sin(angle);
}
context.beginPath();
context.arc(mousedown.x, mousedown.y, radius, 0, Math.PI*2, false);
context.stroke();
if (fillCheckbox.checked)
context.fill();
}
function updateRubberband(loc) {
updateRubberbandRectangle(loc);
drawRubberbandShape(loc);
}
// Guidewires....................................................
function drawHorizontalLine (y) {
context.beginPath();
context.moveTo(0,y+0.5);
context.lineTo(context.canvas.width,y+0.5);
context.stroke();
}
function drawVerticalLine (x) {
context.beginPath();
context.moveTo(x+0.5,0);
context.lineTo(x+0.5,context.canvas.height);
context.stroke();
}
function drawGuidewires(x, y) {
context.save();
context.strokeStyle = 'rgba(0,0,230,0.4)';
context.lineWidth = 0.5;
drawVerticalLine(x);
drawHorizontalLine(y);
context.restore();
}
// Canvas event handlers.........................................
canvas.onmousedown = function (e) {
var loc = windowToCanvas(e.clientX, e.clientY);
e.preventDefault(); // prevent cursor change
saveDrawingSurface();
mousedown.x = loc.x;
mousedown.y = loc.y;
dragging = true;
};
canvas.onmousemove = function (e) {
var loc;
if (dragging) {
e.preventDefault(); // prevent selections
loc = windowToCanvas(e.clientX, e.clientY);
restoreDrawingSurface();
updateRubberband(loc);
if(guidewires) {
drawGuidewires(loc.x, loc.y);
}
}
};
canvas.onmouseup = function (e) {
loc = windowToCanvas(e.clientX, e.clientY);
restoreDrawingSurface();
updateRubberband(loc);
dragging = false;
};
// Controls event handlers.......................................
eraseAllButton.onclick = function (e) {
context.clearRect(0, 0, canvas.width, canvas.height);
drawGrid('lightgray', 10, 10);
saveDrawingSurface();
};
strokeStyleSelect.onchange = function (e) {
context.strokeStyle = strokeStyleSelect.value;
};
fillStyleSelect.onchange = function (e) {
context.fillStyle = fillStyleSelect.value;
};
guidewireCheckbox.onchange = function (e) {
guidewires = guidewireCheckbox.checked;
};
// Initialization................................................
context.strokeStyle = strokeStyleSelect.value;
context.fillStyle = fillStyleSelect.value;
drawGrid('lightgray', 10, 10);
橡皮筋似画图11
原创
©著作权归作者所有:来自51CTO博客作者生而为人我很遗憾的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:扩展绘制-虚线10
下一篇:多边形的绘制与拖动处理12
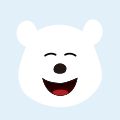
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
模拟橡皮筋
一个模拟橡皮筋的程序。#define _CRT_SECURE_NO_WARNINGS#include <graphics.h>
i++ #define #include -
ios 橡皮筋 禁用
。 前提: 在讲这篇教程之前,如果你对UIBezierPath和CAShapeLayer还不熟悉的话,那需要对这两个方面要做了解,这样才能够看懂代码部分。UIBezierPath可以绘制贝塞尔曲线,那么就需要设置控制点,这里分为三段,其中L3和L2、R1和R2、c和R1
ios 橡皮筋 禁用 动画 UIBezierPath cashaperlayer 贝塞尔曲线 -
直线的“橡皮筋”画法鼠标移动