using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Drawing2D;
using System.Diagnostics;namespace _SCREEN_CAPTURE
{
public partial class MyInput : UserControl
{
private string Content = "";
private int State = 1; //1:编辑 2:选中 3:透明文本
private Font m_font = new Font("宋体", 9);//字体格式
private Color m_color = Color.Blue; //字体颜色
private Point m_downpoint = new Point(); //鼠标按下坐标
private bool DragFlag = false; //拖动标志
Rectangle[] SmallRect = new Rectangle[8];
private int FrameWidth = 6; //边框宽度
private Cursor m_cursor; enum HitDownSquare
{
HDS_NONE = 0,
HDS_TOP = 1,
HDS_RIGHT = 2,
HDS_BOTTOM = 3,
HDS_LEFT = 4,
HDS_TOPLEFT = 5,
HDS_TOPRIGHT = 6,
HDS_BOTTOMLEFT = 7,
HDS_BOTTOMRIGHT = 8
} private HitDownSquare CurrHitPlace;
public MyInput()
{
InitializeComponent();
} void SetRectangles()
{
//定义8个小正方形的范围
Rectangle baseRect = new Rectangle(FrameWidth, FrameWidth, this.Width - FrameWidth * 2, this.Height - FrameWidth * 2);
Size Square = new Size(FrameWidth, FrameWidth);
//左上
SmallRect[0] = new Rectangle(new Point(baseRect.X - Square.Width, baseRect.Y - Square.Height), Square);
//上中间
SmallRect[4] = new Rectangle(new Point(baseRect.X + (baseRect.Width / 2) - (Square.Width / 2), baseRect.Y - Square.Height), Square);
//右上
SmallRect[1] = new Rectangle(new Point(baseRect.X + baseRect.Width, baseRect.Y - Square.Height), Square);
//左下
SmallRect[2] = new Rectangle(new Point(baseRect.X - Square.Width, baseRect.Y + baseRect.Height), Square);
//下中间
SmallRect[5] = new Rectangle(new Point(baseRect.X + (baseRect.Width / 2) - (Square.Width / 2), baseRect.Y + baseRect.Height), Square);
//右下
SmallRect[3] = new Rectangle(new Point(baseRect.X + baseRect.Width, baseRect.Y + baseRect.Height), Square);
//左中间
SmallRect[6] = new Rectangle(new Point(baseRect.X - Square.Width, baseRect.Y + (baseRect.Height / 2) - (Square.Height / 2)), Square);
//右中间
SmallRect[7] = new Rectangle(new Point(baseRect.X + baseRect.Width, baseRect.Y + (baseRect.Height / 2) - (Square.Height / 2)), Square);
} private void MyInput_Paint(object sender, PaintEventArgs e)
{
Graphics gc_main = e.Graphics; if (State == 1)
{
if (m_input.Text == "")
{
this.Size = new Size(4, 14);
}
gc_main.DrawRectangle(new Pen(Color.Blue, 2), 0, 0, this.Width, this.Height);
}
else if (State == 2)
{
gc_main.DrawRectangle(new Pen(Color.LightGray, FrameWidth * 2), 0, 0, this.Width, this.Height); //填充用于调整的边框的内部
Rectangle StringRect = new Rectangle(FrameWidth + 1, FrameWidth + 1, this.Width - FrameWidth * 2, this.Height - FrameWidth * 2);
gc_main.DrawString(Content, m_font, new SolidBrush(m_color), StringRect);
SetRectangles();
gc_main.FillRectangles(Brushes.Black, SmallRect); //填充8个锚点的内部
}
else if (State == 3)
{
Rectangle StringRect = new Rectangle(FrameWidth + 1, FrameWidth + 1, this.Width - FrameWidth * 2, this.Height - FrameWidth * 2);
gc_main.DrawString(Content, m_font, new SolidBrush(m_color), StringRect);
}
} private void MyInput_MouseDown(object sender, MouseEventArgs e)
{
if (e.Button == MouseButtons.Left)
{
m_downpoint = e.Location;
DragFlag = true;
if (State != 2)
{
State = 2;
Invalidate();
}
this.Cursor = m_cursor;
}
} private void MyInput_MouseMove(object sender, MouseEventArgs e)
{
if (State == 2)
{
if (e.Button == MouseButtons.Left && DragFlag)
{
switch (CurrHitPlace)
{
case HitDownSquare.HDS_NONE:
{
Point mousePos = Control.MousePosition; ;
Point p_chart = this.Parent.PointToClient(mousePos);
p_chart.Offset(-m_downpoint.X, -m_downpoint.Y);
if (p_chart.X > 0 && p_chart.X < ((Control)sender).Parent.Width && p_chart.Y > 0 && p_chart.Y < ((Control)sender).Parent.Height)
{
((Control)sender).Location = new Point(p_chart.X, p_chart.Y);
}
}
break;
default:
{
Mouse_Move(this, e); //调整位置或大小
m_downpoint = new Point(e.X, e.Y);
Invalidate();
}
break;
}
}
else
{
Hit_Test(e.X, e.Y); //更新鼠标指针样式
}
}
else
{
this.Cursor = Cursors.Default;
}
} public bool Hit_Test(int x, int y)
{
Point point = new Point(x, y);
Rectangle ControlRect = new Rectangle(0, 0, this.Width, this.Height);
if (!ControlRect.Contains(point))
{
Cursor.Current = Cursors.SizeAll;
return false;
}
else if (SmallRect[0].Contains(point))
{
Cursor.Current = Cursors.SizeNWSE;
CurrHitPlace = HitDownSquare.HDS_TOPLEFT;
}
else if (SmallRect[3].Contains(point))
{
Cursor.Current = Cursors.SizeNWSE;
CurrHitPlace = HitDownSquare.HDS_BOTTOMRIGHT;
}
else if (SmallRect[1].Contains(point))
{
Cursor.Current = Cursors.SizeNESW;
CurrHitPlace = HitDownSquare.HDS_TOPRIGHT;
}
else if (SmallRect[2].Contains(point))
{
Cursor.Current = Cursors.SizeNESW;
CurrHitPlace = HitDownSquare.HDS_BOTTOMLEFT;
}
else if (SmallRect[4].Contains(point))
{
Cursor.Current = Cursors.SizeNS;
CurrHitPlace = HitDownSquare.HDS_TOP;
}
else if (SmallRect[5].Contains(point))
{
Cursor.Current = Cursors.SizeNS;
CurrHitPlace = HitDownSquare.HDS_BOTTOM;
}
else if (SmallRect[6].Contains(point))
{
Cursor.Current = Cursors.SizeWE;
CurrHitPlace = HitDownSquare.HDS_LEFT;
}
else if (SmallRect[7].Contains(point))
{
Cursor.Current = Cursors.SizeWE;
CurrHitPlace = HitDownSquare.HDS_RIGHT;
}
else if (ControlRect.Contains(point))
{
Cursor.Current = Cursors.SizeAll;
CurrHitPlace = HitDownSquare.HDS_NONE;
}
m_cursor = Cursor.Current;
return true;
} public void Mouse_Move(object sender, System.Windows.Forms.MouseEventArgs e)
{
//控件最小为 8x8
if (this.Height < FrameWidth * 2 + 1)
{
this.Height = FrameWidth * 2 + 1;
return;
}
else if (this.Width < FrameWidth * 2 + 1)
{
this.Width = FrameWidth * 2 + 1;
return;
}
switch (this.CurrHitPlace)
{
case HitDownSquare.HDS_TOP:
this.Height = this.Height - e.Y + m_downpoint.Y;
if (this.Height > FrameWidth * 2 + 1)
this.Top = this.Top + e.Y - m_downpoint.Y;
break;
case HitDownSquare.HDS_TOPLEFT:
this.Height = this.Height - e.Y + m_downpoint.Y;
if (this.Height > FrameWidth * 2 + 1)
this.Top = this.Top + e.Y - m_downpoint.Y;
this.Width = this.Width - e.X + m_downpoint.X;
if (this.Width > FrameWidth * 2 + 1)
this.Left = this.Left + e.X - m_downpoint.X;
break;
case HitDownSquare.HDS_TOPRIGHT:
this.Height = this.Height - e.Y + m_downpoint.Y;
if (this.Height > FrameWidth * 2 + 1)
this.Top = this.Top + e.Y - m_downpoint.Y;
this.Width = this.Width + e.X - m_downpoint.X;
break;
case HitDownSquare.HDS_RIGHT:
this.Width = this.Width + e.X - m_downpoint.X;
break;
case HitDownSquare.HDS_BOTTOM:
this.Height = this.Height + e.Y - m_downpoint.Y;
break;
case HitDownSquare.HDS_BOTTOMLEFT:
this.Height = this.Height + e.Y - m_downpoint.Y;
this.Width = this.Width - e.X + m_downpoint.X;
if (this.Width > FrameWidth * 2 + 1)
this.Left = this.Left + e.X - m_downpoint.X;
break;
case HitDownSquare.HDS_BOTTOMRIGHT:
this.Height = this.Height + e.Y - m_downpoint.Y;
this.Width = this.Width + e.X - m_downpoint.X;
break;
case HitDownSquare.HDS_LEFT:
this.Width = this.Width - e.X + m_downpoint.X;
if (this.Width > FrameWidth * 2 + 1)
this.Left = this.Left + e.X - m_downpoint.X;
break;
case HitDownSquare.HDS_NONE:
this.Location.Offset(e.X - m_downpoint.X, e.Y - m_downpoint.Y);
break;
}
} private void MyInput_MouseUp(object sender, MouseEventArgs e)
{
if (State == 2)
{
if (e.Button == MouseButtons.Left)
{
DragFlag = false;
}
else if (e.Button == MouseButtons.Right)
{
InputMenu.Show(PointToScreen(e.Location));
}
}
} private void MyInput_MouseDoubleClick(object sender, MouseEventArgs e)
{
if (e.Button == MouseButtons.Left)
{
m_input.Visible = true;
m_input.Font = m_font;
m_input.Focus();
State = 1;
Invalidate();
}
} private void MyInput_LostFocus(object sender, EventArgs e)
{
if (State == 2)
{
State = 3;
Invalidate();
}
} private Font GetFont(Size s, string P_String)
{
string sFontName = "宋体";
Bitmap _bitmap = new Bitmap(s.Width, s.Height);
Graphics _graphics = Graphics.FromImage(_bitmap);
float fontsize = 0.1f;
for (Size _size = new Size(); _size.Width < s.Width && _size.Height < s.Height; fontsize += 0.1f)
{
Font font_1 = new Font(sFontName, fontsize);
_size = _graphics.MeasureString(P_String, font_1).ToSize();
}
return new Font(sFontName, fontsize - 0.2f);
} private void m_input_TextChanged(object sender, EventArgs e)
{
Graphics gc = this.CreateGraphics(); SizeF TextSize = gc.MeasureString(m_input.Text.ToString(), m_font);
TextSize.Width += FrameWidth * 2 + 2;
TextSize.Height += FrameWidth * 2 + 2;
this.Size = TextSize.ToSize(); Invalidate();
} private void m_input_KeyUp(object sender, KeyEventArgs e)
{
if (e.KeyCode == Keys.Enter)
{
this.Focus();
}
} private void m_input_LostFocus(object sender, EventArgs e)
{
if (m_input.Text == "")
{
Dispose();
return;
}
Content = m_input.Text;
m_input.Visible = false;
State = 3;
Invalidate();
} private void input_delete_Click(object sender, EventArgs e)
{
this.Dispose();
} private void input_property_Click(object sender, EventArgs e)
{
//InputProperties m_pop = new InputProperties();
//m_pop.FontInfo = m_font;
//m_pop.ColorInfo = m_color;
//if (m_pop.ShowDialog() == DialogResult.OK)
//{
// m_font = m_pop.FontInfo;
// m_color = m_pop.ColorInfo;
// Invalidate();
//} EnhanceFontDialog f = new EnhanceFontDialog();
f.SelectedFontColorFromArgb = m_color;
f.SelectedFontStyles = m_font.Style;
f.SelectedFontSize = m_font.Size;
try
{
if (f.ShowDialog(this) == DialogResult.OK)
{
MessageBox.Show(f.SelectedFontColorFromArgb.ToString() + "\n"
+ f.SelectedFontName.ToString() + "\n"
+ f.SelectedFontStyles.ToString() + "\n"
+ f.SelectedFontSize.ToString() + "\n"
+ f.SelectedFontUnit.ToString() + "\n"
, "字体");
m_font = new Font(f.SelectedFontName, f.SelectedFontSize);
m_color = f.SelectedFontColorFromArgb;
Invalidate();
}
}
catch (Exception exc)
{
//handle ur exception
MessageBox.Show("发生错误:" + exc.Message);
}
finally
{
f.Dispose();
}
} private void MyInput_KeyUp(object sender, KeyEventArgs e)
{
if (State == 2)
{
if (e.KeyCode == Keys.Delete)
{
this.Dispose();
}
}
}
}
}
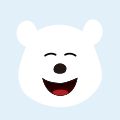
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
使文本框不可编辑【转】
"/> ...
HTML 可编辑 失去焦点 文本框